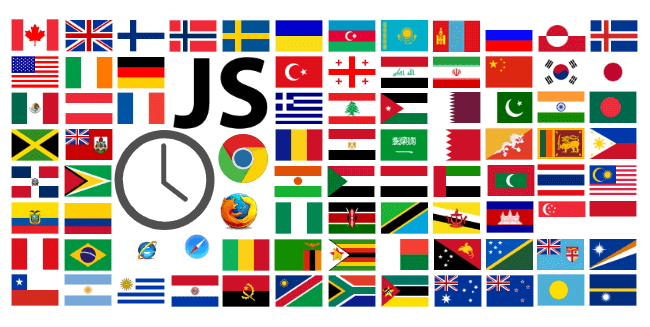
Detecting the country and region of the visiting user on the browser is certainly possible using JavaScript alone, without using any third-party geolocation service such as ipstack. The only consideration: It may not be accurate, and depend on the timezone selected by the user on their system, which can be changed. But since hardly anybody changes their system-auto-detected timezone nowadays (except for testing, which I have shown below), it is a good enough and cheap detection method for non-critical use cases.
[Read More]